|
Python's `json` module is a powerful tool for working with JavaScript Object Notation (JSON) data, a lightweight data interchange format commonly used for transmitting data between a server and a web application, or between different parts of an application. The `json` module provides functions for encoding Python data structures into JSON format and decoding JSON data into Python data structures. Here's an overview of how to use Python's `json` module:
### Encoding (Serialization)
1. **`json.dumps()`**: object (such as a dictionary, list, tuple, or string) into a JSON-formatted string.
```python
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
json_string = json.dumps(data)
print(json_string)
# Output: {"name": "John", "age": 30, "city": "New York"}
```
2. **`json.dump()`**: The `json.dump()` function serializes a Python object and writes hong kong phone number it directly to a file-like object (such as a file handle or `StringIO` object).
```python
with open('data.json', 'w') as f:
json.dump(data, f)
```
### Decoding (Deserialization)
1. **`json.loads()`**: The `json.loads()` function parses a JSON-formatted string and converts it into a Python object.
```python
json_string = '{"name": "John", "age": 30, "city": "New York"}'
data = json.loads(json_string)
print(data)
# Output: {'name': 'John', 'age': 30, 'city': 'New York'}
```
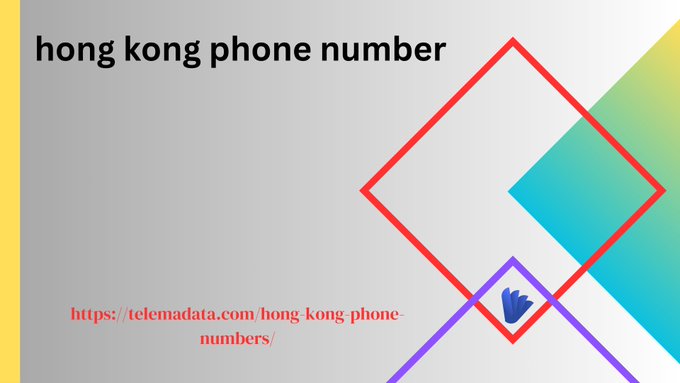
2. **`json.load()`**: The `json.load()` function reads JSON data from a file-like object and parses it into a Python object.
```python
with open('data.json', 'r') as f:
data = json.load(f)
```
### Additional Functionality
1. **Handling Data Types**: The `json` module can handle common Python data types, such as strings, integers, floats, lists, dictionaries, and booleans. Custom objects can also be encoded by providing a custom `default` function or using `json.dumps()` with the `default` parameter.
2. **Error Handling**: The `json` module provides error handling for invalid JSON data using exceptions such as `json.JSONDecodeError` and `json.JSONEncodeError`.
3. **Pretty Printing**: For human-readable JSON output, the `json.dumps()` function accepts parameters like `indent` and `sort_keys` to control formatting.
### Conclusion
Python's `json` module offers a convenient and efficient way to work with JSON data in Python applications. Whether you're serializing Python objects into JSON strings for transmission over the network or deserializing JSON data into Python objects for processing, the `json` module provides a robust and flexible solution for handling JSON data in Python programs.
|
|